Hello World
Your first WADE App. Show some text on the screen.
We are going to start with a classic "Hello World" application. The first thing we need is an entry point for our application. Inside our App function, we should define a member function called init.
The idea of defining a function inside another function may sound strange if you are new to javascript. While in javascript functions can be executed (like in most programming languages), they are also objects that can be instantiated and can have their own properties and members. You could consider App as an object. Now we're adding an init function to that object.
App = function() { this.init = function() { }; }
WADE will recognize this App.init function and call it to initialize our app.
In this function we want to create some text. To do this, we'll create a TextSprite object, like this:
var helloText = new TextSprite('Hello World!');
Now we want our text to appear on the screen: we need to create a SceneObject that contains this text sprite, and tell WADE to add it to the scene.
var helloText = new TextSprite('Hello World!'); var helloObject = new SceneObject(helloText); wade.addSceneObject(helloObject);
Now if you save your test.js file and refresh your app, you should see something like this:
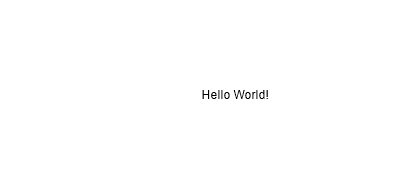
You may be wondering why we did not add the TextSprite directly to the scene: instead, we created a SceneObject that contains our text sprite, then we added that to the scene. This is because TextSprite and SceneObject are conceptually different entities. A TextSprite, like any Sprite object, is just a visual representation of something. Sprites can represent some text (like in this case), or an image (like a character in a game), or some procedural graphics (for example a line chart). SceneObjects, on the other hand, are entities that can be added to the scene: they are objects that exist somewhere in the world and may or may not have a visual representation. When they do, this visual representation is often provided by one or more sprites of any type. SceneObjects can also have their own AI, or behaviors that describe how they react to events (for example, when they're clicked).
Now we could be proud of ourselves for getting some text to appear on the screen, but it still doesn't look very nice. The easiest way to customize the properties of our TextSprite is to pass some additional arguments when it's created:
var helloText = new TextSprite("Hello World!", '32px Verdana', 'red');
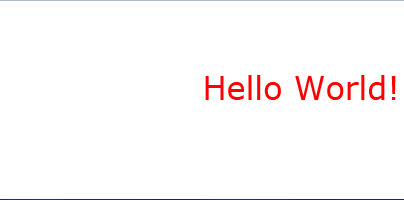
Now it's much nicer, but it's aligned in a strange way. This is because we haven't specified any alignment, and its default alignment is 'left', which means that the left edge of the text sprite is located at the object's position; since we haven't specified an object position either, the object is at the origin (0, 0). So basically, the left edge of the sprite is aligned with the center of the screen. To change this, let's add another parameter for the alignment:
var helloText = new TextSprite("Hello World!", '32px Verdana', 'red', 'center');
Try it right here
Since you're making a web app, and web apps work in web browser, you can try your app right here. In fact, you can edit your app in this box here, then click Update and observe the results live in the box below.
Give it a try, change the color of the text to 'green' and click Update.