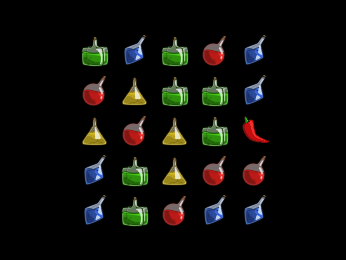
Updated Match3
With Wade 3.7 comes an updated Match3 Editor. The single page had a bit too much data on, so we have split it up into tabs. We’ve also added the brand new events tab, which allows you to execute code on events that the updated match3 behavior now throws.
There are many possible things you can add using events. The onMatch event receives an object which contains a list of blocks that are involved in the match. This data could be used to update scores, counters or bars for specific block types. The handler also allows you to block execution of the next step, which in this case would be removing the involved blocks. To do this, simply return true from the event handler.
Make a static block
Here we will use the new event handlers to make a block which the user cannot manually swap, creating a static block if you will. The block can still move if blocks below it get matched allowing it to fall, but otherwise will remain immobile.
There are multiple ways to do this, but to keep things simple, I'm going to make all green blocks static using the onSwap event.
First we get the scene objects for the two items from the data object. All match3 events receive a "data" object that contains useful information about the event. We need to get the behaviors for "Match3Item" for each item involved in the swap, that is what the first two lines in the image above do.
var item1 = data.square1.getBehavior("Match3Item"); var item2 = data.square2.getBehavior("Match3Item");
In this example, I've decided I want the green items to be static. The "isGreen" function will return true if the tile is green. Using indexOf on the type which includes the "match3_sample_assets" path is a bit questionable. It would be better to check if type equals "match3_sample_assets/green.png" but I didn't want to confuse the example by checking the full name.
var isGreen = function(item) { return item.type.indexOf("green") != -1; }
Finally we call our "isGreen" function for both of our items. If either of them is green, the onSwap handler will return true. In Wade, when you return true inside an event handler, the event will stop propagating. For our match3 behavior, in most cases it is a signal that you want to stop something happening. Returning true from an "onSwap" event handler, will prevent the swap from happening, thus creating our static object.
return isGreen(item1) || isGreen(item2);
I should note that we don't have to make all green tiles static. We could instead make only green tiles that are on odd x co-ordinates immobile, or maybe only tiles around the edges. This is just an example of what you can do with one of the event handlers.
More Events
We have seen how an event handler can be used to change the overall behavior, but there are more. The following events have been added and can all be found in the events tab in the match3 editor.
- onItemRemoved - Triggered when an item is removed from the board.
- onMatch - Triggered when a match occurs, blocks involved are passed in the data object. Returning true will prevent a valid match from occurring.
- onNoMoves - Triggered when there are no valid remaining moves.
- onScore - Triggered whenever the score is updated, score change can be blocked by returning true.
- onSwap - Triggered when a user swipes or drags an item to perform a swap. Returning true will prevent the user from swapping.
All of these events receive a data object describing the event.
Public Functions
In addition to the new events, there are three new public functions in the Match3 Behavior.
- removeItem(x, y) - Removes the item at the provided location. New items will be created and fall to fill the space as normal. Remove Item will not remove the item if the column it belongs to is currently in motion.
- changeItemType(x, y, type) - Changes the type of the item at the given location. If the type is not valid, no change will happen. If changing the type results in a match, it will be treated like any match that occurs after a swap.
- swapItems(x1, y1, x2, y2) - Swaps the items and the provided locations. Identical to the manual swap the user can perform.
