Setup
Set up and get ready to write a WADE App
1.1 Set up
Welcome to the first in a series of short tutorials that will help you get started with WADE.
WADE is an acronym that stands for Web App Development Engine, and is a very powerful tool to create web apps and games that work on a wide variety of devices, because it is a pure HTML5 engine, that requires no browser extensions or external plug-in. HTML5 is the recent update to the HTML standard that has always been used to create web content. There are many new elements in version 5 that make HTML, probably for the first time ever, a viable platform to create complex interactive content. The programming language that is normally used to interact with HTML5 elements is javascript. As you probably know, it's a very powerful dynamic language, that makes it easy to accomplish much with very little typing.
Having said that, it's still very complicated to write efficient HTML5 applications that run everywhere, which is why WADE is extremely useful: it takes care of all the complicated stuff for you, leaving you free to focus on the game or app that you want to make.
An important thing to mention about WADE is that it has a modular architecture: there is a base module, that handles all the basic operations, such as managing 2D objects, moving them around, interacting with them, and giving them some behaviors. More complex operations require more WADE modules, or plug-in's. For many applications you won't need any, but depending on what you are doing, it may be useful to include a WADE module to let the engine manage vector graphics objects, or isometric worlds, or physics for example.
So the first thing that you'll need is a copy of the main WADE module. You can download it here.
It's just a .zip file, so let's have a look at what's inside:
- doc folder. This contains a reference guide with a list of functions, what they do, what all the parameters do, etc.
- wade_xxx.js file. This is the main WADE module itself.
- style.css file. This is a style sheet that is used by browsers to determine how they should render wade applications.#
- index.html file. This is the file that you would open in your browser of choice to run your WADE app.
In fact it would be a good idea to do that right now. Extract the .zip file to a local folder, open your index.html file in a browser, and see what happens. If your browser supports HTML5, you should get a blank (white) page.
Depending on the browser that you're using, you may need to do something else at this point. This is because your files are local (i.e. they are somewhere on your hard drive), and some browsers implement restrictions for local files, for security reasons.
- In Firefox you won't have to do anything.
-
Internet Explorer might show a message box saying that it has blocked some functionality, and you have to click a button that says
Allow Blocked Content
. -
Chrome needs an extra command-line argument to be able to work with local files. Create a shortcut to Chrome, and edit it to add this parameter:
--allow-file-access-from-files
. On a Windows machine, you can do this by right-clicking the Chrome shortcut, selectingProperties
and then adding--allow-file-access-from-files
at the end of the Target field, as shown below:
This is all a bit annoying, but remember that it's only something that you have to do when your files are local. This means that your users won't ever have to do anything like this because, presumably, they are going to access your app from a web server, or from a wrapper object that needs no configuration.
Now create a new, empty javascript file (call it, for example, test.js), and place it in the same folder as everything else. This is going to be your app's main file. For now we'll just leave it empty.
Instead, let's edit the index.html file. You can do this with any text editor. In fact, it's important to note that javascript is a language that doesn't need to be compiled: it can be interpreted, as it is, by web browsers. This means that you won't need any external tools to edit javascript files and to make wade apps. Technically, notepad is all you need.
Doing everything with notepad is a valid option and, importantly, is free. However, as your project grows, you'll need a nicer IDE with advanced functions, code completion, syntax and error highlighting, etc. If you want a free solution, you can get Sublime. If you don't mind spending a little money to get a better working environment, I'd recommend WebStorm. It's free for 30 days, so you should at least try it for now and decide whether it's worth any money later on.
Anyway, open index.html with your favourite tool and you'll notice that it's a small HTML file, with one line of code that is commented out. It looks like this:
$(document).ready(function() { // wade.init('yourApp.js'); });
You want to uncomment that line of code, and point it to your newly created app file. So it will look something like this:
$(document).ready(function() { wade.init('test.js'); });
By doing this, we're initializing WADE, telling it to load a main app file called test.js. For test.js to be recognized as a valid main app file by WADE, it needs to be a valid javascript file with no errors, and it needs to define a variable called App that is a function.
So let's edit test.js now, and add these lines to it:
App = function() { }
App is the function that represents our WADE application.
Now is a good time to save index.html and test.js and refresh the browser window with your app. If everything is working correctly, you should still see a blank page with no error messages.
An alternative way to host WADE apps is to use PromptJS. This is the method preferred here at Clockwork Chilli. Once downloaded and installed, hosting a WADE app is easy. Simply navigate to the folder containing your app via the cd (change directory) command, and type "server 1234". This will host your app on port 1234, though you can choose any port you wish. To run the app, open up a browser of your choice and in the url bar type "localhost:1234" without quotes.
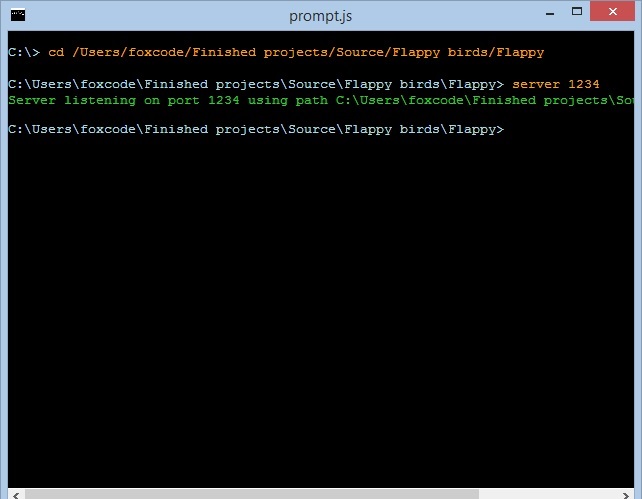
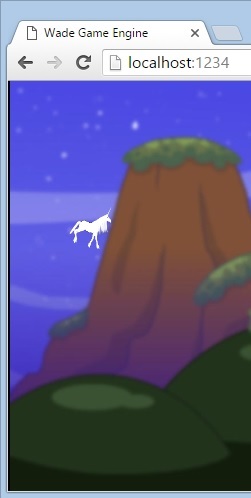
We're now ready to start using WADE.